7 minutes of reading
The Beginner's Guide to Server-Side Rendering
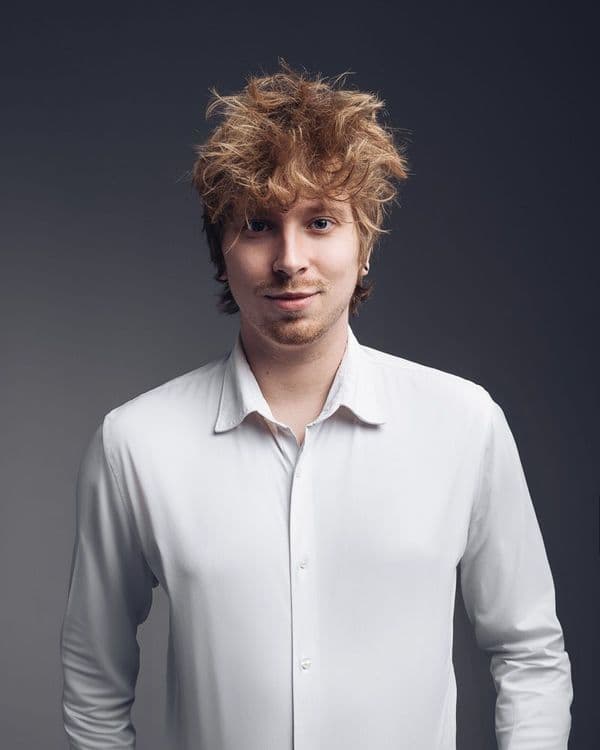
Maksymilian Konarski
20 September 2024
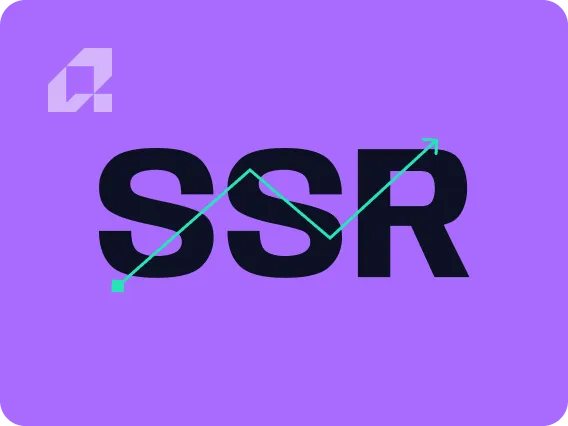
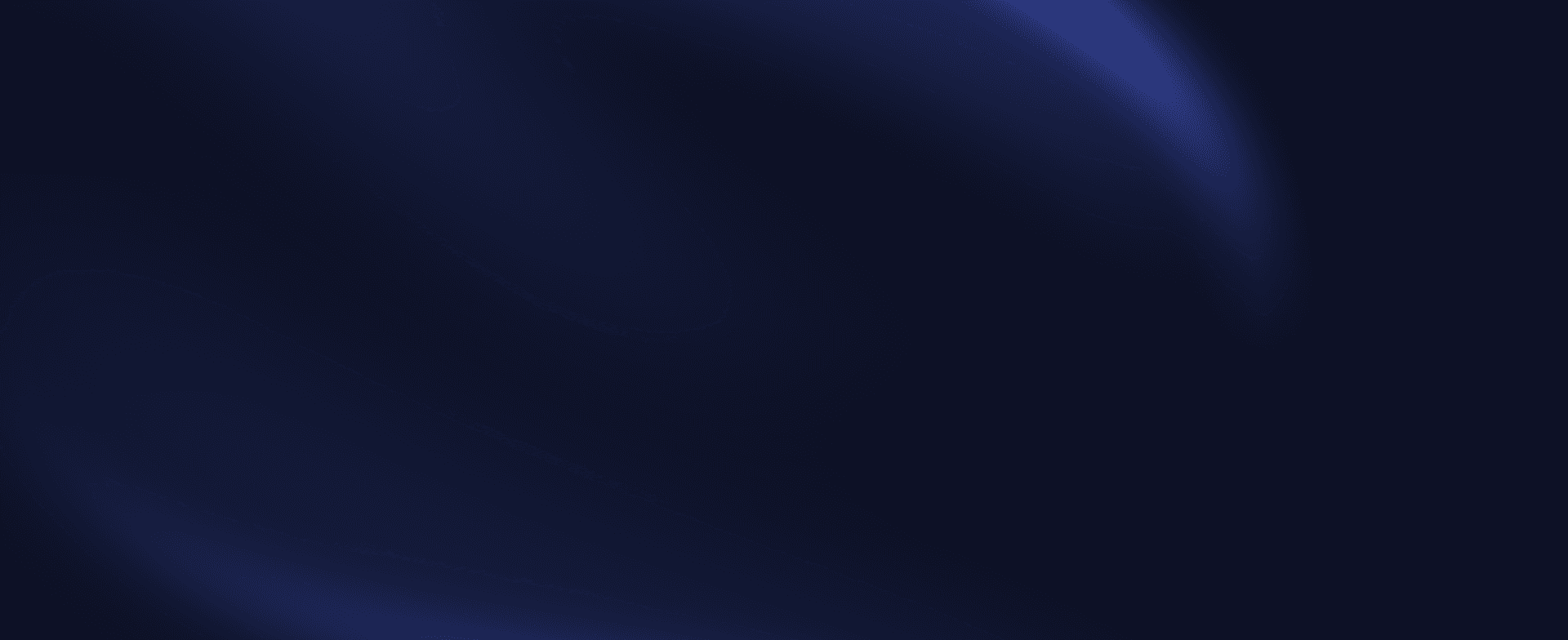
Server-side rendering, or SSR, is a web development technique in which the webpage is generated on the server instead of rendering directly to the client’s browser. The biggest advantage of SSR web pages is that they ensure quick transitions and initial page load, elevating the user experience and increasing user satisfaction. Unlike client-side rendering or CSR, which sends full-page HTML for each request, SSR sends a minimal HTML document. While SSR improves the website’s performance and SEO, CSR makes the web page more interactive. SSR is usually used by content-heavy websites, SEO optimization projects, target users with slow and unreliable networks, etc.
How Server-Side Rendering Works
SSR is basically the process starting with a user requesting a website and the web page becoming interactable. At first, the user enters a request for the website they want to interact with. Once the web address is entered, the server processes the request and creates an HTML file. Then, the browser renders the HTML and sends Javascript to the browser, and the websites become fully interactive and ready for the client to use. As the initial web page uses a fully rendered HTML file, it requires minimal Javascript, decreasing wait time for the user.
Advantages of SSR
Improved SEO
With server-side rendering, the HTML is fully rendered within the system, and the content is readily indexed by the time it reaches the search engine bot. Because the search engine crawlers understand the web content and index better, it ensures SEO benefits for the web page by enhancing its discoverability. As a result, it improves the website’s search engine ranking. Moreover, as the HTML is fully rendered, SSR ensures metadata, such as content titles, descriptions, social media tags, and other SEO attributes, are met, which is also beneficial for improving a website’s SEO.
Performance Benefits
The server-side rendering pipeline has a minimal dependency on Javascript, which is why it doesn’t require a long waiting time for the web page to load and become intractable. Moreover, SSR only updates the Javascript that needs updating, which speeds up the page transition time. As a result, users with a slow internet connection can easily access these websites. It is also reliable for users with limited power-processing devices.
Since the HTML is rendered before it goes to the client’s browser, it also reduces client-side execution workload, lowering server strain. Furthermore, its ability to offer a fast response throughout the process ensures optimized source usage. It also helps reduce the file sizes downloaded to the device via the browser.
User Experience
SSR does not require downloading or running any application code. As a result, it reduces the web page’s load time and improves content visibility. Also, using the SSR technique with Next.js or Nuxt.js framework is one of the best ways to enhance interactive experience and seamless navigation. Compared to CSR, SSR also offers improved accessibility for slow internet and device users.
Setting Up SSR
Choosing a Framework
The most crucial step in setting up SSR is choosing the right framework. Some popular ones are Next.js, Nuxt.js, Angular Universal, SvelteKit, etc. However, choosing the right framework depends on your content, project type, user type, etc. You should compare the frameworks to understand their pros and cons and find what fits your project best. SSR has a vast community and offers wider learning resources. Joining these communities for support and discussing with the experts can also help you find the best framework.
Basic Setup
Here is the basic setup breakdown for server-side rendering (SSR):
Step 1: Initial Project Setup
Create a new project directory for your SSR application and cd into it. Then, initialize the new project using a package manager like npm, yarn, or npm init—y. It is also essential to choose a framework or library, as discussed above.
Step 2: Installing Dependencies
Make sure you have the required dependencies, such as react, redux, react-dom, react-redux, express, etc., installed.
Step 3: Configuring Server
You need to set up an Express server to handle the server-side rendering. To serve the components, you need to create an Express application, define entry points, specify routes, configure middleware, etc..
Step 4: Setting up Routes
Define routes for your SSR application to handle incoming requests. Find edits at the bottom and make sure the app.get('*') is below each of your declared routes. Using libraries like NextUs or react-router helps you navigate and handle route changes of your application.
Step 5: Client-Side Hydration
You can elevate the user experience using client-side react to "hydrate" the server-rendered HTML. And it is done according to your component type. For example, you need to use ReactDOM.hydrate() in the pre-rendered HTML on the client side to render the React components.
Step 6: Testing Setup
You must test the setup occasionally to ensure it works as it should. If there is a bug or management issue, you must resolve it using debugging tools or getting help from SSR communities.
Common Challenges and Solutions
Performance Optimization
There are several challenges regarding SSR performance optimizations and one of them is caching strategies. Caching occurs when there is a load of requests and inefficiency. Sometimes, caching the system or memory also leads to performance issues. Implementing a multi-tier caching system is the best way to resolve the issue.
Another similar issue happens when the server becomes overwhelmed by an incoming request. And it can be fixed by implementing a load balancer and distributing the request across multiple servers to reduce server load. The SSR performance also gets interrupted due to the lack of code splitting or lazy loading, which slows down the initial page loading and increases unnecessary download time. Using tools like Webpack, Browserify, and Rollup to reduce bundle size and improve efficient loading.
Debugging Issues
SSR technology is often prone to bugs, such as client/server mismatch, flickering due to rendering, incorrect React SSR markup, error logging, etc. But they can be resolved using debugging tools and Microsoft's Visual Studio Code is one of them. Other such tools include Chrome DevTools and general SSR debugging approaches. You can also browse forums and find community support for the best debugging solutions.
Security Concerns
HTTP requests involve using user data, which can lead to data and privacy breaches. Hackers or attackers can access user data using XSS or cross-site scripting. That is why developers must implement high data transmission, access authentication, and encryption communication. Ensuring user data and system security also involves updating frameworks, tools, and strategies regularly.
Advanced SSR Techniques
Static Site Generation
To improve your SSR performance, you can apply some advanced techniques to improve performance. One such technique is the use of SSG or Static Site Generation. Using templates with content plugged into them, SSG creates several HTML files for each web page ahead of time. As a result, the server doesn’t need to do anything when a user visits the website. While SSR generates web pages on request and in real-time, SSR builds it before that and serves pre-built HTML files. Popular SSG tools are Next.js, Nuxt.js, Gatsby, Jekyll, Eleventy, Hugo, Pelican, etc. SSG approach is highly suitable for static content and high-traffic sites.
Hybrid Approaches
One of the best ways to improve a website’s visibility and SEO is to combine SSR with CSR. This ensures faster page load and makes the website more interactive, increasing user satisfaction. Also, you can send Javascript for the interactive parts, and react will hydrate those parts only, implementing partial hydration. The Incremental Static Regeneration (ISR) approach also improves the website performance, ensures real-time updates, and is cost-effective.
Popular Tools and Libraries
Next.js for React: Next.js works with React to generate server-side rendering technology. It improves performance, makes websites user-friendly, brings consistency, and increases user satisfaction.
Nuxt.js for Vue: The routes of SSR with the Nuxt.js framework use the Vue.js method. It renders the data and HTML inside the template, which helps send general HTML files to the clients.
Angular Universal: This Javascript is built by Google, and using this tool users can build SSR apps.
SvelteKit: It sends HTML to the client, where it will be hydrated. Developers now tend to use it because it helps avoid framework bloating.
Node.js: Node.js offers a unified language for front and backend SSR development.
Express.js: It can handle several gate requests simultaneously on a single web page.
GraphQL: It optimizes data fetching and improves overall performance.
Summary
In the digital landscape and fast-changing web development world, server-side rendering is widely adopted as it offers faster web page load, reduces wait time, and improves website SEO. However, choosing the right SSR framework or web application architecture is also vital. After choosing the framework, install the dependencies, configure the server, and set up the routes. Along with many challenges, SSR is pushing the boundaries and shaping the future of web development as it is compatible even with slow internet and weak mobile devices.
Conclusion
Since SSR is a powerful technique that highly influences website performance, it is recommended for all web developers. This technique can also make the website more user-friendly and generate more traffic. You can learn more about SSR through Next.js Documentation, Nuxt.js Documentation, Angular Universal Guide on SSR, Vite.js, etc. You can also join various SSR online communities for further discussion and guidelines.
What can we do for you?
Web Application Development
Build Lightning-Fast Web Apps with Next.js
AI Development
Leverage AI to create a new competitive advantage.
Process Automation
Use your time more effectively and automate repetitive tasks.
Digital Transformation
Bring your company into the 21st century and increase its efficiency.
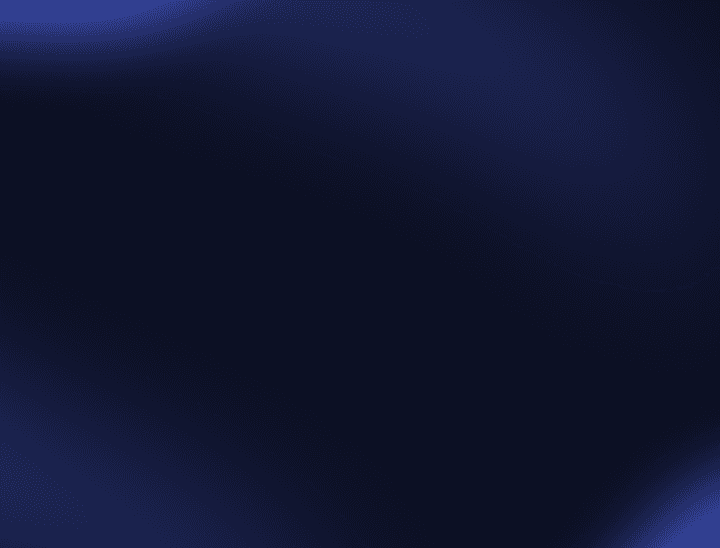
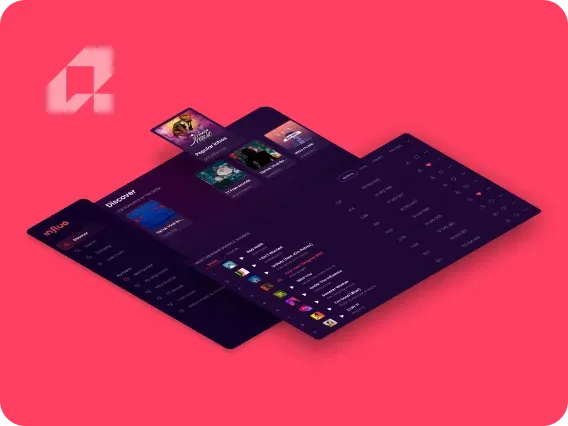
Automating Software Testing: Benefits and Best Practices
Explore the perks and key methods of automating software testing. Streamline your processes and boost efficiency with expert tips.
8 minutes of reading
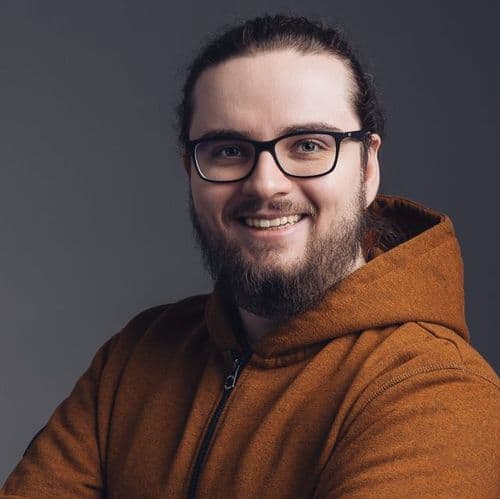
Michał Kłak
06 May 2024
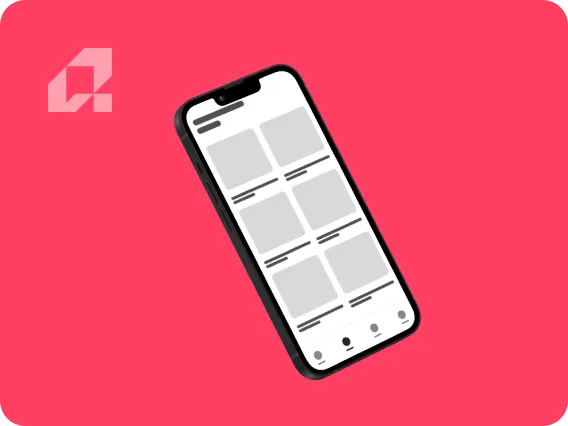
Mobile app development cost breakdown - how much does it cost to make a mobile app?
Learn what influences mobile app development costs, from platform choice and functionality to user research, security, and team expertise.
12 minutes of reading
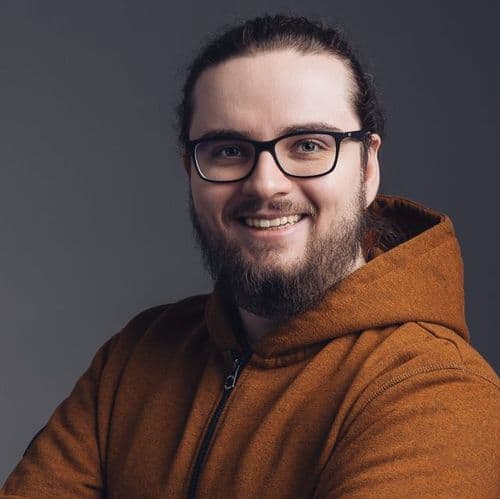
Michał Kłak
23 December 2024
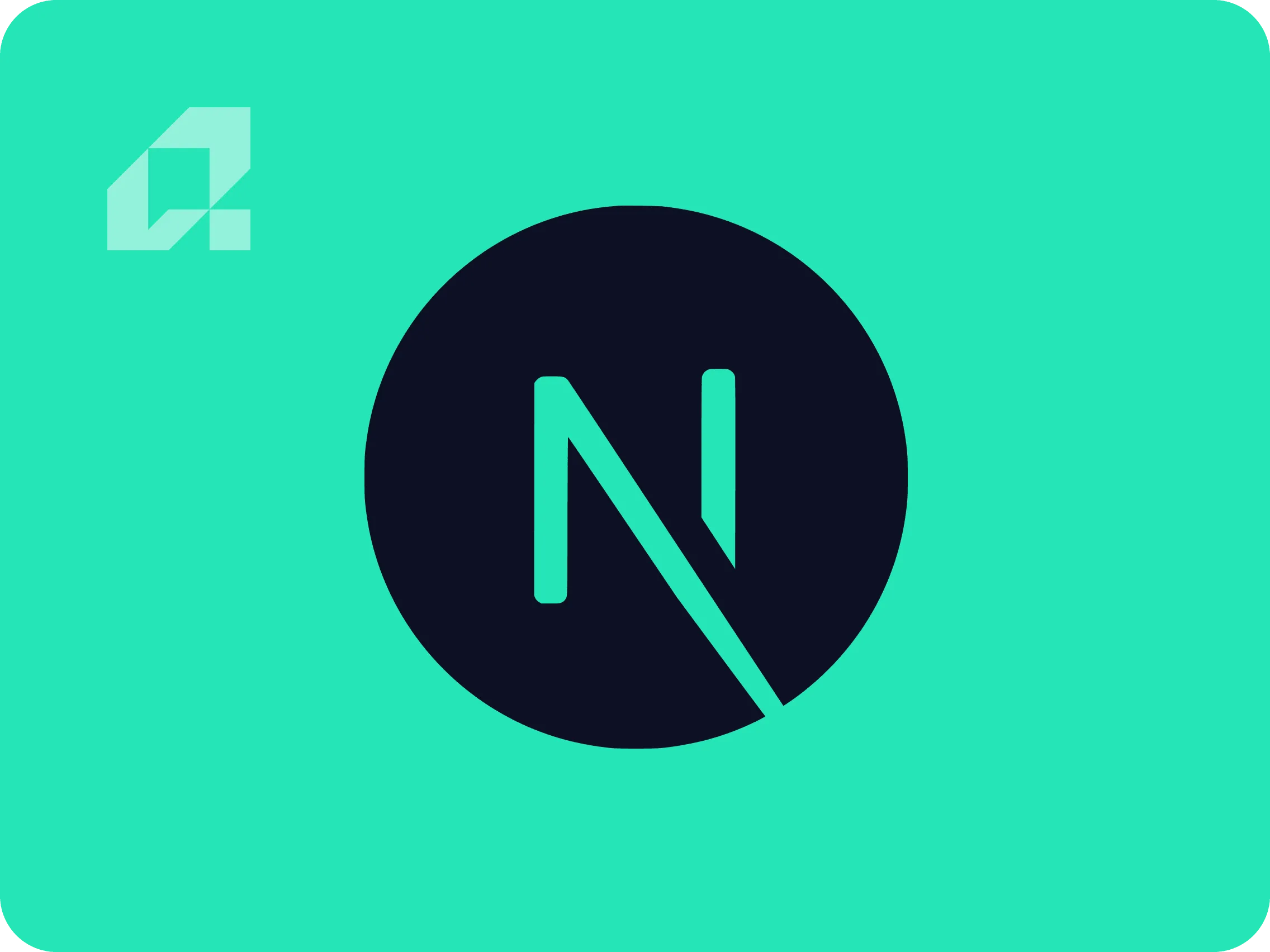
Next.js - what is this framework? Advantages and disadvantages of this technological solution
Discover Next.js advantages like SEO and SSR, and explore challenges such as complex integrations and learning curve.
12 minutes of reading
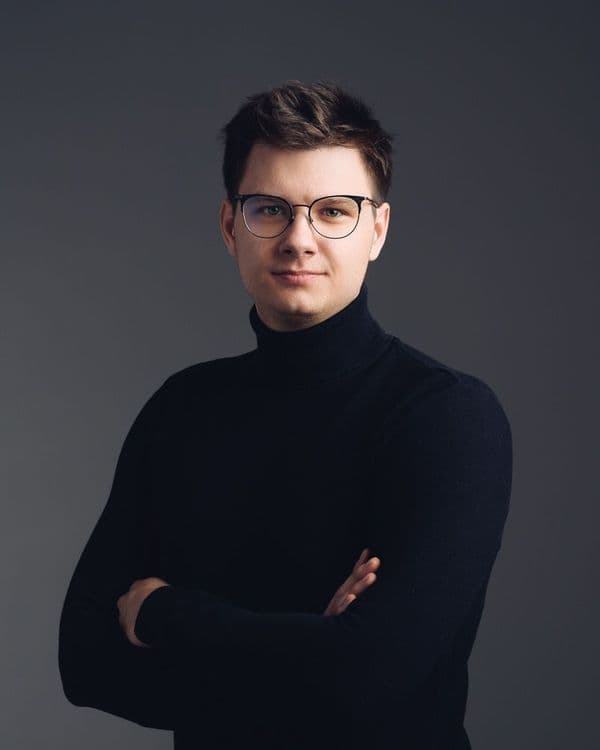
Oskar Szymkowiak
24 September 2024
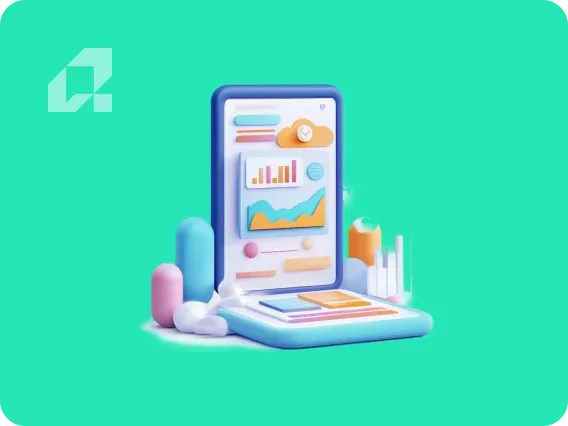
Best technologies for web application development in 2024
Top Web Development Technologies for 2024
6 minutes of reading
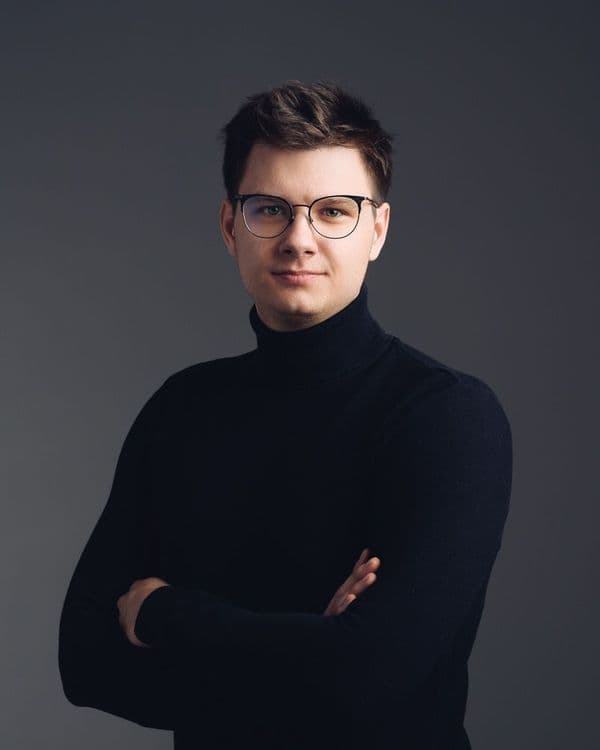
Oskar Szymkowiak
05 February 2024